In programming, lists are perhaps as useful a data structure as arrays. What are lists, how to create them? How to work with lists in Python? You will learn about this from our article.
What are lists in Python?
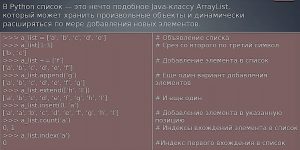
Lists can partially be identified with arrays, but the difference and advantage of listings (otherwise they are also called listings) is that they can combine different data types. That is, the listing opens up more possibilities for storing any sequence of objects. A variable, called a list, contains a reference to a structure in memory that contains references to alternative structures.
A list in Python is an ordered collection of objects of mixed types that can be modified and whose objects may differ.
What does it mean? Let’s take a look at the definition in detail.
The size of the listing can be changed, reduced, new lines added to it. You can also change the entire structure of the list. Keep in mind that every time a method in a list is used, the original list is changed, not the copy.
For greater clarity, you can think of a listing in Python as a list of products that need to be bought in a store. If, when making a shopping plan, all the necessary items are located one below the other, and each of them has its own line, then the listing in Python contains all the elements separated by commas and in square brackets so that Python can understand that a list is indicated here. The elements are enclosed in quotation marks. This is a mandatory condition, because each element is a separate line.
Ways to create a list
Moving on to the classic example, let’s create a list that we will use and modify in the future. There are several ways to generate listings.
One of them is the application built-in function list( ). To do this, you need to process any object that can be iterated (a string, a tuple, or an existing list). In this case, a string.
Here’s what happens in the end:
>>> list('list') ['c', 'n', 'i', 'c', 'o', 'to']
The second example shows that lists can contain an unlimited number of very different objects. Also, the listing can remain empty.
>>> s = [] # Empty list >>> l = ['s', 'p', ['isok'], 2] >>> s [] >>> l ['s', 'p' , ['isok'], 2]
The next, third, way of forming listings is the so-called listing generator.
The listing generator is a syntactic construct for creating listings. It is similar to the for loop.
>>> c = [c * 3 for c in 'list'] >>> c ['lll', 'iii', 'sss', 'ttt']
It can also be used to create more voluminous structures:
>>> c = [c * 3 for c in 'list' if c != 'i'] >>> c ['lll', 'sss', 'ttt'] >>> c = [c + d for c in 'list' if c != 'i' for d in 'spam' if d != 'a'] >>> c ['ls', 'lp', 'lm', 'ss', 'sp', 'sm', 'ts', 'tp', 'tm']
However, this generation method is not always efficient when compiling multiple listings. Therefore, it is advisable to use a for loop to generate listings.
If you need to refer to any element from the list, then indexes are used. Each element has its own index.
The index is the number of the element in the list.
If you want to fill the listing with repeating, identical elements, the * symbol is used. For example, you need to add three identical numbers to the listing: [100] * 3.
Listing functions
functions – this is perhaps the main advantage of Python over other programming languages. Basic built-in functions can be applied to lists.
Consider the most popular of them:
- list(range( )) – if the task is to create a sequential list, then the range function is used. This function has the following forms:
- range(end). It is used when it is necessary to create a listing from zero to a finite number.
- range(start, end). Both start and end numbers are specified.
- range(start, end, step). The step parameter specifies the selection characteristic. For example, if you need to select every fifth number from a sequence from 1 to 21, then the resulting listing will look like: [10,15, 20].
The range function can significantly reduce the amount of code.
- flax (list) – allows you to find out how many elements are in the list.
- sorted(list, [key]) – sorts the objects in the list in ascending order.
- max (list) – returns the largest element.
- min (list) – opposite function – allows you to return the element with the minimum value.
You can also use other built-in functions:
- list(tuple) – Converts a tuple object to a list.
- sum(list) – sums all elements in the list if all values are numbers, applies to both integers and decimals. However, she doesn’t always get it right. If there is a non-numeric element in the list, the function will throw an error: “TypeError: unsupported operand type(s) for +: ‘int’ and ‘str'”.
Listing Methods
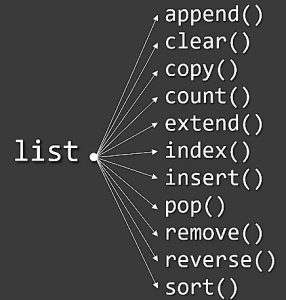
Let’s go back to our list of items to buy in the store and call it shoplist:
shoplist = []
Next, consider the listing methods:
- append(item) – with its help, you can add an element to the list. In this case, the new element will be at the end.
Let’s fill our new listing with the right products:
shoplist.append(bread)
shoplist.append(milk)
- list.extend(A) – adds “list to list“. This feature saves time as you can add multiple items at the same time. Let’s say we already have a listing of fruits, we need to add them to the main list.
shoplist.extend(fruits)
- insert(index, item) – inserts on the element with the specified index the specified value before the specified index.
- lcount(item) – shows the number of repetitions of the element.
- list.remove(item) is the opposite function list.append (x). It can be used to remove any element. If the selected item is not in the list, an error is reported.
- pop([index]) – removes the selected element and returns it in the same way. If the element is not specified, then the last element is removed from the list.
- sort([key]) – puts the elements in the listing in ascending order, but you can also specify a function.
- index(item) – shows the index of the first selected element.
- You can expand the list, that is, mirror all its elements, using the method reverse(list). The last element becomes the first, the penultimate element becomes the second, and so on.
- A copy of the list is created with the command copy(list).
- deepcopy(list) – deep copying.
- Remove all listing elements using the method clear(list).
It is worth noting that listing methods differ from string methods in that they immediately change the list, that is, there is no need to return the result of execution.
>>> l = [1, 2, 3, 5, 7] >>> l.sort() >>> l [1, 2, 3, 5, 7] >>> l = l.sort() >>> print(l) None
The following is an example of working with lists:
>>> a = [66.25, 333, 333, 1, 1234.5] >>> print(a.count(333), a.count(66.25), a.count('x')) 2 1 0 >>> a.insert(2, -1) >>> a.append(333) >>> a [66.25, 333, -1, 333, 1, 1234.5, 333] >>> a.index(333) 1 >>> a.remove(333) >>> a [66.25, -1, 333, 1, 1234.5, 333] >>> a.reverse() >>> a [333, 1234.5, 1, 333, -1, 66.25] >>> a.sort() >>> a [-1, 1, 66.25, 333, 333, 1234.5]